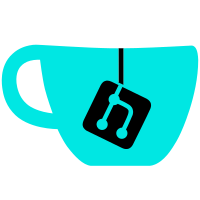
* Add `html-parser-api` and `html-parser-impl` modules * Add `HtmlEmptyTagReplacement` * Implement Appendable and CharSequence in SpannableBuilder * Renamed library modules to reflect maven artifact names * Rename `markwon-syntax` to `markwon-syntax-highlight` * Add HtmlRenderer asbtraction * Add CssInlineStyleParser * Fix Theme#listItemColor and OL * Fix task list block parser to revert parsing state when line is not matching * Defined test format files * image-loader add datauri parser * image-loader add support for inline data uri image references * Add travis configuration * Fix image with width greater than canvas scaled * Fix blockquote span * Dealing with white spaces at the end of a document * image-loader add SchemeHandler abstraction * Add sample-latex-math module
61 lines
1.5 KiB
Java
61 lines
1.5 KiB
Java
package ru.noties.markwon.il;
|
|
|
|
import android.support.annotation.Nullable;
|
|
|
|
public class DataUri {
|
|
|
|
private final String contentType;
|
|
private final boolean base64;
|
|
private final String data;
|
|
|
|
public DataUri(@Nullable String contentType, boolean base64, @Nullable String data) {
|
|
this.contentType = contentType;
|
|
this.base64 = base64;
|
|
this.data = data;
|
|
}
|
|
|
|
@Nullable
|
|
public String contentType() {
|
|
return contentType;
|
|
}
|
|
|
|
public boolean base64() {
|
|
return base64;
|
|
}
|
|
|
|
@Nullable
|
|
public String data() {
|
|
return data;
|
|
}
|
|
|
|
@Override
|
|
public String toString() {
|
|
return "DataUri{" +
|
|
"contentType='" + contentType + '\'' +
|
|
", base64=" + base64 +
|
|
", data='" + data + '\'' +
|
|
'}';
|
|
}
|
|
|
|
@Override
|
|
public boolean equals(Object o) {
|
|
if (this == o) return true;
|
|
if (o == null || getClass() != o.getClass()) return false;
|
|
|
|
DataUri dataUri = (DataUri) o;
|
|
|
|
if (base64 != dataUri.base64) return false;
|
|
if (contentType != null ? !contentType.equals(dataUri.contentType) : dataUri.contentType != null)
|
|
return false;
|
|
return data != null ? data.equals(dataUri.data) : dataUri.data == null;
|
|
}
|
|
|
|
@Override
|
|
public int hashCode() {
|
|
int result = contentType != null ? contentType.hashCode() : 0;
|
|
result = 31 * result + (base64 ? 1 : 0);
|
|
result = 31 * result + (data != null ? data.hashCode() : 0);
|
|
return result;
|
|
}
|
|
}
|