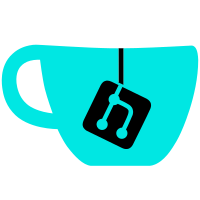
* Add `html-parser-api` and `html-parser-impl` modules * Add `HtmlEmptyTagReplacement` * Implement Appendable and CharSequence in SpannableBuilder * Renamed library modules to reflect maven artifact names * Rename `markwon-syntax` to `markwon-syntax-highlight` * Add HtmlRenderer asbtraction * Add CssInlineStyleParser * Fix Theme#listItemColor and OL * Fix task list block parser to revert parsing state when line is not matching * Defined test format files * image-loader add datauri parser * image-loader add support for inline data uri image references * Add travis configuration * Fix image with width greater than canvas scaled * Fix blockquote span * Dealing with white spaces at the end of a document * image-loader add SchemeHandler abstraction * Add sample-latex-math module
42 lines
1.0 KiB
Java
42 lines
1.0 KiB
Java
package ru.noties.markwon.il;
|
|
|
|
import android.support.annotation.NonNull;
|
|
import android.support.annotation.Nullable;
|
|
import android.text.TextUtils;
|
|
import android.util.Base64;
|
|
|
|
public abstract class DataUriDecoder {
|
|
|
|
@Nullable
|
|
public abstract byte[] decode(@NonNull DataUri dataUri);
|
|
|
|
@NonNull
|
|
public static DataUriDecoder create() {
|
|
return new Impl();
|
|
}
|
|
|
|
static class Impl extends DataUriDecoder {
|
|
|
|
@Nullable
|
|
@Override
|
|
public byte[] decode(@NonNull DataUri dataUri) {
|
|
|
|
final String data = dataUri.data();
|
|
|
|
if (!TextUtils.isEmpty(data)) {
|
|
try {
|
|
if (dataUri.base64()) {
|
|
return Base64.decode(data.getBytes("UTF-8"), Base64.DEFAULT);
|
|
} else {
|
|
return data.getBytes("UTF-8");
|
|
}
|
|
} catch (Throwable t) {
|
|
return null;
|
|
}
|
|
} else {
|
|
return null;
|
|
}
|
|
}
|
|
}
|
|
}
|