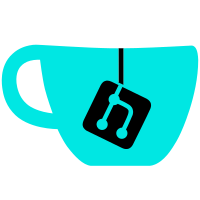
Since the headers for cpu, memory and load functions are virtually the same for all platforms, I've decided to move them into common/ dir and do some refacotring: * removed per-platform header files * implemented get_cpu_count() function across all platforms. We are using it cpu on every platform, yet not on every one this was implemented as a separate function. * removed platform detection through preprocessor from main: we don't need this there anymore, since the headers are common for all platforms. CMake will handle setting of correct source files for us now. * Unified used defines for CPU states across all platforms and made linux use them. Added some platform detection to cpu.h in order to set them correctly across the platforms. * moved getsysctl.h to common/ dir, since it's used on Net and Free BSD, and thus become a common include.
84 lines
2.4 KiB
C++
84 lines
2.4 KiB
C++
/* vim: tabstop=2 shiftwidth=2 expandtab textwidth=80 linebreak wrap
|
|
*
|
|
* Copyright 2012 Matthew McCormick
|
|
* Copyright 2015 Pawel 'l0ner' Soltys
|
|
*
|
|
* Licensed under the Apache License, Version 2.0 (the "License");
|
|
* you may not use this file except in compliance with the License.
|
|
* You may obtain a copy of the License at
|
|
*
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
*
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS,
|
|
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
* See the License for the specific language governing permissions and
|
|
* limitations under the License.
|
|
*/
|
|
|
|
#include <unistd.h> // usleep
|
|
|
|
#include <sys/sysctl.h>
|
|
#include <sys/types.h>
|
|
|
|
#include "error.h"
|
|
#include "cpu.h"
|
|
|
|
uint8_t get_cpu_count()
|
|
{
|
|
int cpu_count = 1; // default to 1
|
|
int mib[2] = { CTL_HW, HW_NCPU };
|
|
size_t len = sizeof( cpu_count );
|
|
|
|
if( sysctl( mib, 2, &cpu_count, &len, NULL, 0 ) < 0 )
|
|
{
|
|
error( "sysctl: error getting cpu count" );
|
|
}
|
|
|
|
return cpu_count;
|
|
}
|
|
|
|
float cpu_percentage( unsigned int cpu_usage_delay )
|
|
{
|
|
int cpu_ctl[] = { CTL_KERN, KERN_CPTIME };
|
|
|
|
u_long load1[CP_STATES];
|
|
u_long load2[CP_STATES];
|
|
|
|
size_t size = sizeof( load1 );
|
|
|
|
// get cpu times
|
|
if( sysctl( cpu_ctl, 2, &load1, &size, NULL, 0 ) < 0 )
|
|
{
|
|
error( "sysctl: error getting initial cpu stats" );
|
|
}
|
|
|
|
usleep( cpu_usage_delay );
|
|
|
|
// update cpu times
|
|
if( sysctl( cpu_ctl, 2, &load2, &size, NULL, 0 ) < 0 )
|
|
{
|
|
error( "sysctl: error getting updated cpu stats" );
|
|
}
|
|
|
|
// Current load times
|
|
unsigned long long current_user = load1[CP_USER];
|
|
unsigned long long current_system = load1[CP_SYS];
|
|
unsigned long long current_nice = load1[CP_NICE];
|
|
unsigned long long current_idle = load1[CP_IDLE];
|
|
// Next load times
|
|
unsigned long long next_user = load2[CP_USER];
|
|
unsigned long long next_system = load2[CP_SYS];
|
|
unsigned long long next_nice = load2[CP_NICE];
|
|
unsigned long long next_idle = load2[CP_IDLE];
|
|
// Difference between the two
|
|
unsigned long long diff_user = next_user - current_user;
|
|
unsigned long long diff_system = next_system - current_system;
|
|
unsigned long long diff_nice = next_nice - current_nice;
|
|
unsigned long long diff_idle = next_idle - current_idle;
|
|
|
|
return static_cast<float>( diff_user + diff_system + diff_nice ) /
|
|
static_cast<float>( diff_user + diff_system + diff_nice + diff_idle ) *
|
|
100.0;
|
|
}
|